Building an Image Grid
In building the new Chaffey Building Group website, the design included an image grid as seen below:
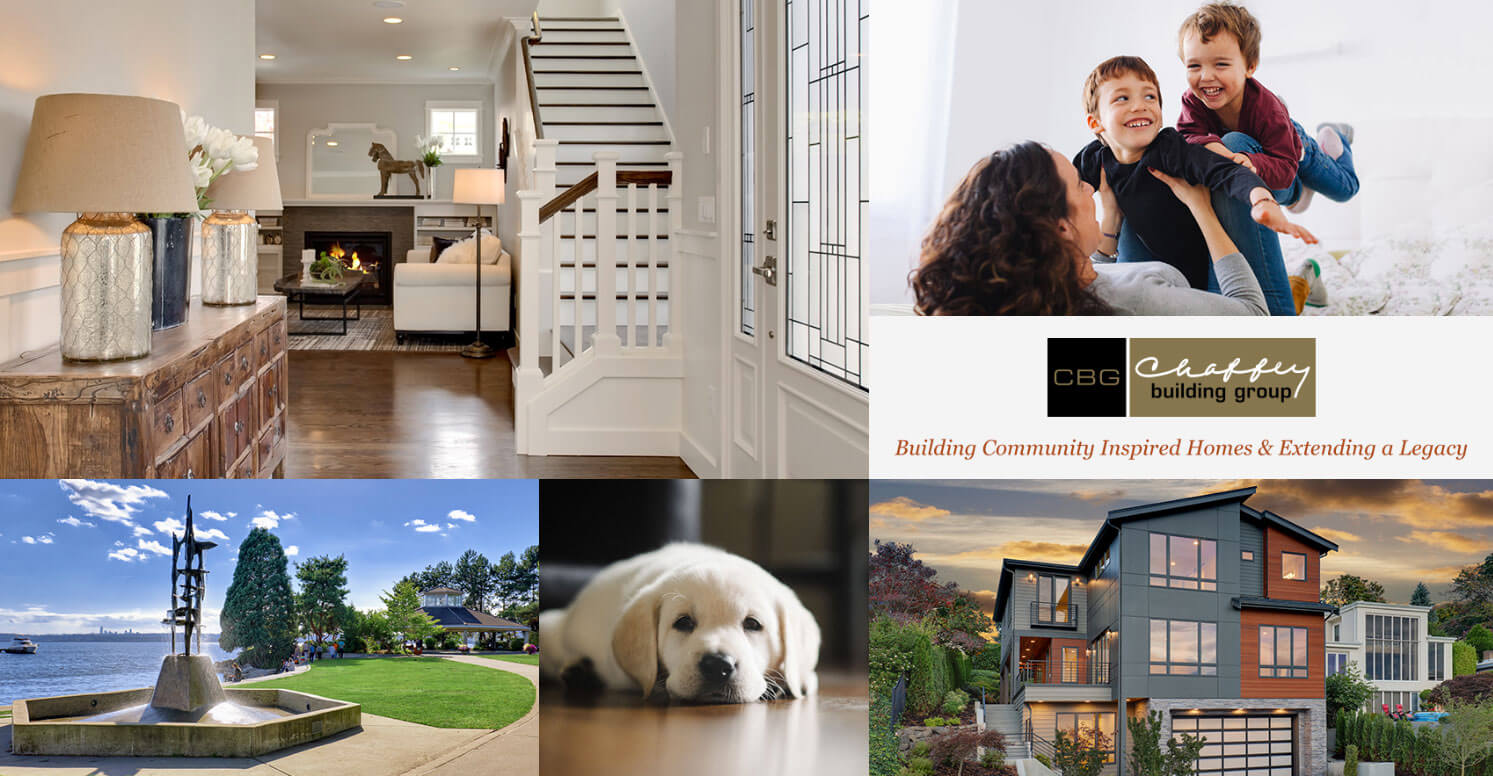
I thought about whether to use CSS Grid or Flexbox for the layout. I decided on Flexbox as each image requires a specific percentage of width and really only needs the one dimension, which Flexbox is best suited for.
In my work, I typically run a Grunt task use the SASS pre-processor for CSS along with post-css autoprefixer. This allows me to write simple rules without worrying about the correct format for older versions of Flexbox.
So, the SASS as written here:
1 2 3 4 5 | .entry-content { display:flex; flex-wrap:wrap; margin-top:0; } |
Turns into:
1 2 3 4 5 6 7 8 9 10 | .entry-content { display: -webkit-box; display: -webkit-flex; display: -ms-flexbox; display: flex; -webkit-flex-wrap: wrap; -ms-flex-wrap: wrap; flex-wrap: wrap; margin-top: 0; } |
One we have the flexbox container, the next thing to handle are the images or in this case the sections that contain the images. Since I like to code CSS or SASS mobile first, the initial image section settings would just default to a width of 100%. I do set the order property so that the image with the logo appears first in the mobile view. Images are already set to display:block and here they given a height of 100% to ensure no gaps. Note the selector nesting as we are using the SASS preprocessor. And we do not have to worry about older or browser specific versions of order as post-css takes care of this.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | .image1 { order:2; img { min-height:100%; } } .image2 { order:1 } .image3 { order:3; img { min-height:100%; } } .image4 { order:4; img { min-height:100%; } } .image5 { order:4; img { min-height:100%; } } |
Once the screen is wide enough, the two smaller images appear side by side:
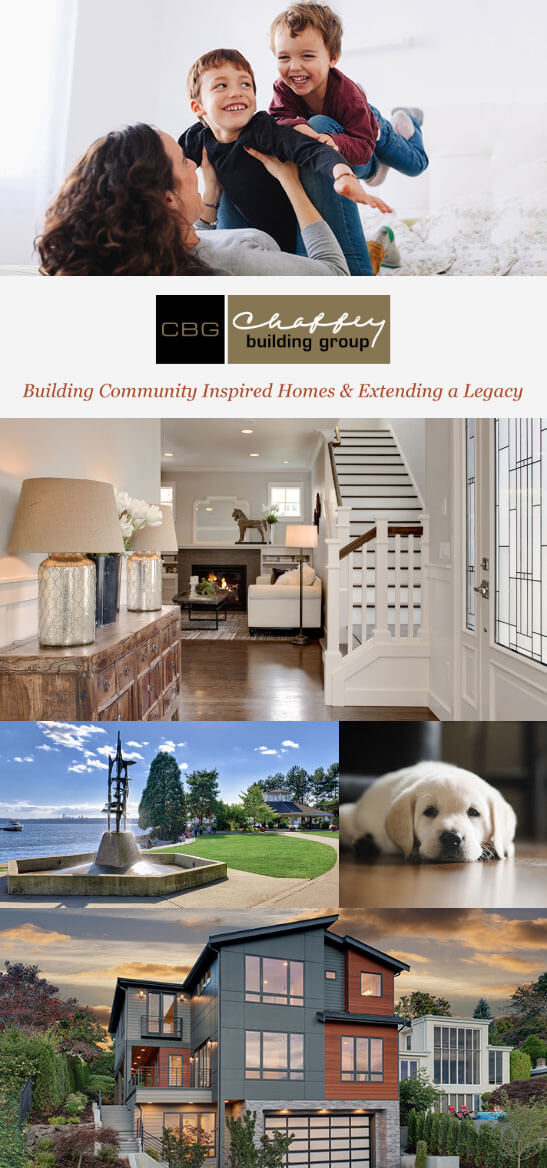
1 2 3 4 5 6 | .image4 { width:38%; } .image3 { width:62%; } |
Finally, we have the code for the large screen version:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | section { margin:0; &.logo { order:2; } } .image1 { order:1; width:58.117647%; } .image2 { order:2; } .image2, .image5 { width:41.882353%; } .image3 { width:36.058824%; } .image4 { width:22.058824%; } |
The one “gotcha” I discovered in the process was Safari on the mac and IOS. It seemed to wrap the second image section rather than keeping them on one row. After digging around on how to address this only for Safari, I ended up with this code:
1 2 3 4 5 6 7 8 9 | @media not all and (min-resolution:.001dpcm) { @supports (-webkit-appearance:none) and (stroke-color:transparent) { .site-home-content .image1 { margin-left:-2px; } }} |
Finally, here is the HTML for the image grid:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | <div class="entry-content"> <section class="image1"> <img class="home-image" src="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Interior-top-left.jpg" srcset="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Interior-top-left.jpg" 988w,="" https:="" chaffeybuildinggroup.com="" wp-content="" uploads="" 2018="" 07="" interior-top-left-300x166.jpg="" 300w,="" interior-top-left-768x424.jpg="" 768w="" alt="CBG Chaffey Building Group Home Page Image"> </section> <section class="image2 logo"> <img class="home-image" src="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/RET-Lifestyle-top-right-1.jpg" srcset="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/RET-Lifestyle-top-right-1.jpg" 712w,="" https:="" chaffeybuildinggroup.com="" wp-content="" uploads="" 2018="" 07="" ret-lifestyle-top-right-1-300x152.jpg="" 300w="" alt="CBG Chaffey Building Group Home Page Image"> <h1><img class="home-image" src="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Home-composite-4.png" srcset="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Home-composite-4.png" 712w,="" https:="" chaffeybuildinggroup.com="" wp-content="" uploads="" 2018="" 07="" home-composite-4-300x78.png="" 300w="" alt="CBG Chaffey Building Group Home Page Logo"></h1> </section> <section class="image3"> <img class="home-image" src="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Kirkland-bottom-left-1.jpg" srcset="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Kirkland-bottom-left-1.jpg" 613w,="" https:="" chaffeybuildinggroup.com="" wp-content="" uploads="" 2018="" 07="" kirkland-bottom-left-1-300x165.jpg="" 300w="" alt="CBG Chaffey Building Group Home Page Image"> </section> <section class="image4"> <img class="home-image" src="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/puppy-1.jpg" srcset="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/puppy-1.jpg" 375w,="" https:="" chaffeybuildinggroup.com="" wp-content="" uploads="" 2018="" 07="" puppy-1-300x270.jpg="" 300w="" alt="CBG Chaffey Building Group Home Page Image"> </section> <section class="image5"> <img class="home-image" src="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Exterior.jpg" srcset="https://chaffeybuildinggroup.com/wp-content/uploads/2018/07/Exterior.jpg" 712w,="" https:="" chaffeybuildinggroup.com="" wp-content="" uploads="" 2018="" 07="" exterior-300x142.jpg="" 300w="" alt="CBG Chaffey Building Group Home Page Image"> </section> </div> |
For more posts on modern css please see Modern CSS – part 2 and Modern CSS – part 3.
-0 Comments